You've Reached the Center of the Internet
It's a blog
ESP8266 with Web Server using Arduino IDE
I saw a talk about internet of things today. Got me thinking about the ESP8266. I've messed with them a little bit before, but I never really got one working, I don't think.
I gave it another try tonight and I've got a simple web server running on one. Here's how I did it:
First, I bought one of these usb to serial adapters. Previously I had been trying to use and Arduino as the usb to serial adapter. I think this could probably work, but it added that unnecessary level of complexity and doubt that can get in the way. Also, I only had an Uno on hand, so I was using 5V. The ESP is a 3.3V device and I wasn't doing any level shifting or anything. Again, maybe it would have just worked.
Next, I wired the ESP up to the serial adapter like this:
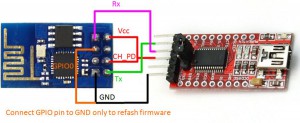
That GPIO0 pin functions both as an I/O pin, and as the pin that tells the microcontroller to get ready to have its firmware flashed. To write new firmware, you have to hold that pin down, I'm told.
Next, I installed a new copy of the Arduino IDE. I had been using the one in the Ubuntu repository (which is an older version) and I was having trouble. Now I'm using 1.6.6. To install the ESP8266 package, I basically followed the "Using Git Version" section of this Github.
I cd'd into the new Arduino directory and then followed these instructions:
cd hardware
mkdir esp8266com
cd esp8266com
git clone https://github.com/esp8266/Arduino.git esp8266
cd esp8266/tools
python get.py
Then I restarted the IDE.
I chose the HelloServer example from the ESP8266WebServer folder. It looks like this:
#include <ESP8266WiFi.h>
#include <WiFiClient.h>
#include <ESP8266WebServer.h>
#include <ESP8266mDNS.h>
const char* ssid = "{YOUR SSID HERE}";
const char* password = "{YOUR PASSWORD HERE}";
ESP8266WebServer server(80);
const int led = 13;
void handleRoot() {
digitalWrite(led, 1);
server.send(200, "text/plain", "hello from esp8266!");
digitalWrite(led, 0);
}
void handleNotFound(){
digitalWrite(led, 1);
String message = "File Not Found\n\n";
message += "URI: ";
message += server.uri();
message += "\nMethod: ";
message += (server.method() == HTTP_GET)?"GET":"POST";
message += "\nArguments: ";
message += server.args();
message += "\n";
for (uint8_t i=0; i<server.args(); i++){
message += " " + server.argName(i) + ": " + server.arg(i) + "\n";
}
server.send(404, "text/plain", message);
digitalWrite(led, 0);
}
void setup(void){
pinMode(led, OUTPUT);
digitalWrite(led, 0);
Serial.begin(115200);
WiFi.begin(ssid, password);
Serial.println(");
// Wait for connection
while (WiFi.status() != WL_CONNECTED) {
delay(500);
Serial.print(".");
}
Serial.println(");
Serial.print("Connected to ");
Serial.println(ssid);
Serial.print("IP address: ");
Serial.println(WiFi.localIP());
if (MDNS.begin("esp8266")) {
Serial.println("MDNS responder started");
}
server.on("/", handleRoot);
server.on("/inline", [](){
server.send(200, "text/plain", "this works as well");
});
server.onNotFound(handleNotFound);
server.begin();
Serial.println("HTTP server started");
}
void loop(void){
server.handleClient();
}
Next, I selected "Generic ESP8266 Module" as the board and USBasp as the programmer, and uploaded. After the upload was successful, I unplugged GPIO0 and let it float.
I used my router's configuration page to figure out which IP had been assigned to my ESP8266. It was easy to find it in the list of connected devices, since it was called ESP_something.
I pointed my browser at the ESP's IP and it worked!